728x90
반응형
Pandas
데이터 처리와 분석을 위한 파이썬 라이브러리이다.
R의 data.frame을 본떠서 설계한 DataFrame이라는 데이터 구조를 기반으로 만들어졌다.
엑셀의 스프레드시트와 비슷한 테이블 형태를 가진다.
SQL처럼 테이블에 쿼리나 조인을 수행할 수 있다.
SQL, 엑셀 파일, CSV 파일 같은 다양한 파일과 DB에서 읽어들일 수 있다.
Pandas 설치
pip install pandas
Pandas 데이터 구조
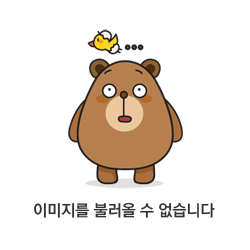
Data Structure | Dimensionality | Spreadsheet Analog |
Series | 1D | Column |
DataFrame | 2D | Single Sheet |
Panel | 3D | Multiple Sheet |
Series
Python의 목록과 유사한 1차원 데이터를 모델링하는데 사용된다.
Series 객체에는 색인 및 이름을 포함하여 몇 비트 더 많은 데이터가 있다.
특징
- 레이블 또는 데이터의 위치를 지정한 추출이 가능하다.
- 산술 연산이 가능하다.
import pandas as pd
s1 = pd.Series([10, 7, 1, 22], index=['a', 'b', 'c', 'd'], name='Name')
>>
a 10
b 7
c 1
d 22
Name: Name, dtype: int64
Indexing Summary
Method | When to Use |
속성 엑세스 | 이름이 유효한 경우 단일 색인 이름에 대한 값 가져오기 속성 이름 |
인덱스 엑세스 | 이름이 아닌 경우 단일 이름에 대한 값 가져오기 / 설정 유효한 속성 |
.iloc | 인덱스 위치 또는 위치별로 값 가져오기 / 설정(반 개방슬라이드 간격) |
.loc | 색인 레이블로 값 가져오기 / 설정(슬라이스 폐쇄 간격) |
.lat | 인덱스 위치별로 numpy 배열 결과 가져오기 / 설정 |
.at | 인덱스 레이블로 numpy 배열 결과 가져오기 / 설정 |
import pandas as pd
s1 = pd.Series([10, 7, 1, 22], index=['a', 'b', 'c', 'd'], name='Name')
s1.iloc[0]
>> 10
s1.loc['a']
>> 10
s1.iloc[0:3]
>>
a 10
b 7
c 1
Name: Name, dtype: int64
s1.loc['a':'c']
>>
a 10
b 7
c 1
Name: Name, dtype: int64
DataFrame
행과 열에 레이블을 가진 2차원 데이터이다.
열마다 다른 형태를 가질 수 있다.
테이블형 데이터에 대해 불러오기, 데이터 쓰기가 가능하다.
DataFrame끼리 여러 가지 조건을 사용한 결합 처리가 가능하다.
크로스 집계가 가능하다.
import pandas as pd
ldata1 = [('kim', 20, 180), ('lee', 40, 160), ('oh', 10, 190)]
df = pd.DataFrame(ldata1, columns=['name', 'age', 'height'])
>>
name age height
0 kim 20 180
1 lee 40 160
2 oh 10 190
DataFrame 생성 후 개별적으로 각 열 정의
import pandas as pd
df = pd.DataFrame()
df['Name'] = ['Jacky' , 'Steve']
df['Age'] = [35, 14]
df['Driver'] = [True, False]
>>
Name Age Driver
0 Jacky 35 True
1 Steve 14 False
새로운 열 또는 행 추가
import pandas as pd
import numpay as np
df = pd.DataFrame(np.arange(6).reshape(2,3),
index=['one','two'],
columns=['seoul', 'busan', 'incheon'])
>>
seoul busan incheon
one 0 1 2
two 3 4 5
# 새로운 열 추가
df['suwon'] = pd.Series([10, 20], index=['one', 'two'])
>>
seoul busan incheon suwon
one 0 1 2 10
two 3 4 5 20
# 새로운 행 추가
df.loc['three'] = pd.Series([100, 200, 300, 400], index=['seoul', 'busan', 'incheon', 'suwon'])
>>
seoul busan incheon suwon
one 0 1 2 10
two 3 4 5 20
three 100 200 300 400
728x90
반응형
'Python > Pandas' 카테고리의 다른 글
[Pandas] 데이터 정렬하기 (0) | 2022.04.26 |
---|---|
[Pandas] 데이터프레임 병합 (0) | 2022.04.26 |
[Pandas] 데이터프레임 연결 (0) | 2022.04.26 |
[Pandas] apply 함수, applymap 함수, map 함수 (0) | 2021.05.10 |
[Pandas] Pandas-Profiling (0) | 2021.04.12 |